How to Deploy a React Application on GitHub: Step-by-Step Guide
Imagine this: you’ve spent days—or maybe weeks—perfecting your React project.
It could be a personal portfolio to highlight your skills, a demo to impress a potential client, or simply a fun project you want to share with friends.
You’re ready to show it off, but here’s the question: how do you host it online without paying for server space or setting up complex configurations?
This is where GitHub Pages comes in, almost like a backstage pass that gets your project straight onto the stage with minimal effort.
For developers, GitHub Pages is a free, hassle-free way to share static websites, and that includes your React applications.
From project portfolios to quick demos, GitHub Pages gives your work an audience—you only need to push changes to your repository, and your live site is updated automatically.
What is GitHub Pages and Why Use it for Deployment?
GitHub Pages is a free hosting service provided by GitHub that’s perfect for deploying static websites.
It’s designed to serve HTML, CSS, and JavaScript files directly from a GitHub repository, which means it’s ideal for any project that doesn’t require server-side processing—and yes, that includes React applications!
When you compile a React app, it’s converted into static files that GitHub Pages can effortlessly host.
In this guide, we’ll go through each step of getting your React application online.
We’ll start by setting up your React app, move on to configuring essential fields in package.json, and finally deploy your app so it’s live on GitHub Pages.
With this approach, you’ll be able to share your work with a single URL, making deployment as straightforward as a quick Git push.
Prerequisites
Before starting, make sure you have:
- A GitHub Account: Sign up if you don’t have one.
- Node.js and npm Installed: Download them from nodejs.org. To verify, use `node -v` and `npm -v` to check their versions.
- A Basic Understanding of Git.
Host a React Page on GitHub: Step-by-Step Guide
Step 1: Set Up Your React App
What does this step mean?
Creating a React app is the first step to getting your project ready for deployment.
Even if you already have a project, it’s important to double-check the basics to avoid issues later.
If you don’t have a React app yet, you can quickly create one with the following command:
Replace my-app with your project’s name. Once it’s ready, test it locally:
Open http://localhost:3000 in your browser to verify that it’s running.
[Note: npx automatically uses the latest version of create-react-app, which eliminates the need for global installation.]
Example:
Imagine you’re building a portfolio to showcase your skills.
Running ‘npm start’ to preview your app can help you spot any issues locally before they’re visible online.
It’s like checking your appearance in a mirror before a big presentation—better to catch those quirks early on!
Step 2: Configure GitHub Repository
What does this step mean?
This step involves creating a space on GitHub where your project will live.
It’s like setting up a “home” for your app on the internet.
Linking your local files to GitHub ensures any changes are updated online with minimal effort.
▶️Go to GitHub, click the ‘+’ icon, and select ‘New repository’.
▶️Name your repository (use your project’s name for consistency).
▶️Choose ‘Public’ and click ‘Create repository’.
After creating the repository, link it to your project:
Replace ‘yourusername’ and ‘your-repository’ with your GitHub username and repository name.
The command ‘git init’, is necessary only if the project hasn’t been initialized as a Git repository.
[Note: Ensure the main branch is set as the default branch (some repositories default to master)].
Step 3: Install gh-pages
What does this step mean?
The gh-pages package is a deployment helper.
It streamlines the process of building and pushing your project files to a specific branch where GitHub can host them.
Installing this package is essential to make deployment efficient.
[Note: gh-pages must be installed in the project directory (and not globally)].
To install gh-pages, run:
Example:
For any developer juggling multiple projects, gh-pages is like having a direct line to production.
Instead of juggling FTP clients or different servers, it allows you to deploy with a single command.
This package is a lifesaver, especially when working on projects where quick updates are crucial.
Step 4: Update package.json for Deployment
What does this step mean?
In this step, we tell GitHub where to find your project files and set up scripts for an easy deployment process.
Without this configuration, your app might not display correctly after deployment.
Open your package.json file and make the following changes:
1. Add a homepage Field:
This field ensures that all paths in your app point correctly when hosted on GitHub.
[Note: For the "homepage" field, the URL format must match the repository's case sensitivity (GitHub is case-sensitive for repository names)].
2. Add Deployment Scripts:
Under "scripts", add:
Now, whenever you run npm run deploy, it will build your app and push it to GitHub Pages.
Imagine you’re setting up a storefront. Without signs (like homepage), customers (or users) might get lost looking for your “aisles” (pages).
This simple line makes sure visitors land where they’re supposed to, avoiding any dreaded “Page Not Found” errors.
Step 5: Deploy the App
What does this step mean?
This is the moment of truth where your project goes live.
Running npm run deploy builds your app and pushes it to the gh-pages branch on GitHub, making it accessible online.
To deploy, run:
After it’s done, GitHub will serve your app from the gh-pages branch, making it live at the URL you specified in the homepage field.
Example:
It’s like putting a sign on a storefront: “Open for Business!”
Running this command is your grand opening to the world.
After deployment, anyone with the link can access your app.
Step 6: Verify Deployment on GitHub Pages
What does this step mean?
After deploying, it’s crucial to double-check that everything works as expected.
This step ensures that your app’s layout, functionality, and links appear correctly.
To verify:
▶️Go to the ‘Settings’ tab in your GitHub repository.
▶️Scroll down to ‘GitHub Pages’.
▶️Select the ‘gh-pages’ branch as your source.
Visit the live URL:
Replace ‘yourusername’ and ‘your-repository’ with your GitHub username and repository name.
Example:
Consider this your quality check—like walking through a newly launched website or app.
A quick scan of each page helps you catch any final bugs and see your project from a fresh perspective.
This step might reveal issues that weren’t obvious during local testing, ensuring users see the best version.
Step 7: Enable HTTPS for Secure Access
What does this step mean?
Enabling HTTPS ensures that your application is served over a secure connection, providing a layer of trust and data encryption for your users.
Without HTTPS, browsers might flag your site as “Not Secure,” which could discourage visitors.
To enable HTTPS:
▶️ Go to your GitHub repository and click on the ‘Settings’ tab.
▶️ Under ‘GitHub Pages’, scroll down to the HTTPS section.
▶️ Check the box labeled ‘Enforce HTTPS’.
Example:
Think of HTTPS as adding a lock to your storefront door.
It not only secures your application but also gives users confidence that their data is safe when interacting with your site.
Enforcing HTTPS is a small step that makes a big difference in ensuring a professional and secure user experience.
Step 8: Optimize Your Build for Better Performance
What does this step mean?
Before deploying your app, optimizing your build ensures it loads faster and performs better for end users.
A smaller build size translates to quicker load times, especially important for mobile and slower network connections.
To optimize your build:
▶️Open your terminal and ensure you are in the root directory of your React app.
▶️Run the following command to build your app in production mode:
Verify that the build folder contains the optimized static files ready for deployment.
Example:
Imagine you’re preparing for a big event. Optimizing your build is like rehearsing your performance to ensure everything runs smoothly.
An optimized build not only enhances performance but also leaves a lasting impression on visitors by delivering a seamless user experience.
Troubleshooting Tips
Sometimes things don’t go perfectly, and that’s okay.
This section addresses common issues and provides solutions to keep your deployment running smoothly.
- Blank Page: Ensure the homepage field in package.json is correct.
For the "Blank Page" issue, double-check the build folder and the structure of static files.
- 404 Errors: If using react-router, use HashRouter or add a 404.html redirect to your public folder.
Example:
Imagine launching a demo only to see a blank screen.
This can be as unsettling as setting up a pop-up store and having no visitors!
With these quick fixes, you can tackle common roadblocks and avoid “blank screen” surprises on launch day.
Advanced Tips for Managing Deployment
These optional tips are helpful if you want more control over your GitHub Pages site.
Features like custom domains or GitHub Actions for automation add polish and convenience.
- Custom Domain: Add a custom domain by creating a CNAME file in the public folder with your domain name.
- Automated Deployments: Currently, the deployment branch is "gh-pages".
To change it, go to your repository settings, select Pages, and choose the desired branch from the Source dropdown.
Use GitHub Actions to automate deployments for the configured branch (e.g., "gh-pages" or another branch).
Example:
Think of custom domains as choosing a “prime” address. Instead of yourusername.github.io, having www.yourproject.com adds a professional touch to your site.
And with automated deployments, updates are instant—no more manually deploying each time you push code!
Conclusion
You’ve now deployed your React app on GitHub Pages!
This free and easy hosting solution is perfect for sharing work, gathering feedback, or running a live demo.
Hosting on GitHub Pages not only showcases your skills but provides an accessible way to connect with a broader audience.
Try deploying more projects, and don’t hesitate to share your GitHub Pages link with colleagues or potential employers.
Still have questions?
Send an email to archana@leadwalnut.com OR
Book a FREE consultation with an expert developer here.
Get in touch - Our team has developed scalable solutions for enterprises and has a Crunch rating of 4.9⭐.
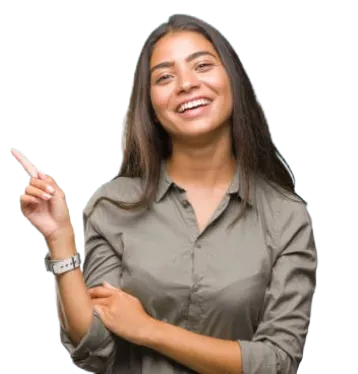
Experience coding prowess firsthand. Choose CodeWalnut to build a prototype within a week and make your choice with confidence.
Accelerate your web app vision with CodeWalnut. In just a week, we'll shape your idea into a polished prototype, powered by Vercel. Ready to make it real? Choose us with confidence!
Dreaming of a powerful web app on Heroku? Let CodeWalnut bring it to life in just one week. Take the leap and trust us to deliver with confidence!