Spring Boot: Best Practices for Scalable Applications
Hey there, tech enthusiasts! 👋
We all know about building applications with a Java backend using Spring Boot. It’s a popular choice for crafting dynamic and robust enterprise-level products. But have you ever wondered how large-scale applications are developed using Spring Boot while ensuring code efficiency, clean practices, performance optimization, full monitoring, and seamless deployment?
In this guide, we’ll explore the entire process of building high-quality applications with Spring Boot. You’ll learn how to maintain clean and efficient code, optimize for performance, implement thorough monitoring, and achieve flawless deployment. Get ready to elevate your development skills and create enterprise-level applications that truly stand out. 🚀
Setting Up Your Spring Boot Project
Let's set up the Spring Boot project. Spring Boot is a framework that simplifies the development of Java applications by providing default configurations and features. We will leverage this
Steps to Create a Spring Boot Application:
Now, your Spring Boot application is up and running, ready to serve requests from the frontend.
Building a Clean and Efficient Codebase
Creating clean and efficient code is essential for maintaining a scalable and high-performing application. In this section, we'll cover:
- Structuring Your Spring Boot Projects: Organizing your codebase to enhance readability and manageability.
- Writing Clean Code: Adopting best practices to ensure your code is easy to understand, maintain, and extend. also using tools like Lombok to minimize repetitive code.
- Implementing Effective Testing: Using tools like JUnit to write robust unit tests and Mockito for mocking dependencies, ensuring your code is reliable and bug-free.
Structuring the Project
Structuring a project is crucial for maintaining clean, readable, and manageable code. There are various ways to structure a Spring Boot project, each catering to different needs and preferences. Below, we will explore effective strategies for organizing your Spring Boot application to ensure optimal structure and maintainability.
A well-structured Spring Boot project ensures that all the components are organized logically, making the codebase easier to navigate and maintain. Here are some recommended structures:
Writing Clean Code: Best Practices
Clean code is crucial for maintaining a high-quality codebase. It enhances readability, reduces bugs, and makes your code easier to maintain and extend. Here, we'll explore best practices for writing clean code in Spring Boot projects.
In this refactored example:
- UserService is now responsible only for user creation.
- UserValidator is responsible for validating the user.
- NotificationService is responsible for sending notifications.
This separation of concerns makes each class easier to understand, test, and maintain. If there is a change in the validation logic, it will only affect the UserValidator class without impacting UserService or NotificationService. This adherence to SRP leads to a more modular and maintainable codebase.
2. Use Dependency Injection
- Dependency Injection (DI) is a design pattern used to implement IoC (Inversion of Control), allowing the creation of dependent objects outside of a class and providing those objects to the class in different ways.
- In Spring Boot, DI helps manage your application's dependencies automatically, reducing the need for manual instantiation and configuration of objects.
- Benefits include improved testability, easier refactoring, and more maintainable code.
Example:
Let's consider a UserService that depends on a UserRepository to interact with the database.
Without Dependency Injection:-
Problems:
Tight Coupling: UserService directly creates instances of UserRepository, NotificationService, and AuditService, making it difficult to test and change these dependencies.
Hard to Mock: Testing UserService would require real instances of UserRepository, NotificationService, and AuditService, making unit tests dependent on these implementations.
With Dependency Injection:-
- In this example, UserService no longer creates an instance of UserRepository. Instead, UserRepository is injected into UserService via the constructor.
- The @Autowired annotation tells Spring to automatically inject an instance of UserRepository when creating the UserService bean.
- This approach makes UserService loosely coupled to UserRepository, enhancing testability and flexibility. You can easily swap out UserRepository with a different implementation, such as a mock repository for unit testing.
3. Use Lombok for Boilerplate Code
- Lombok is a Java library that helps reduce boilerplate code by automatically generating getters, setters, constructors, toString(), equals(), and hashCode() methods using annotations.
- By using Lombok, you can make your code more concise and readable, and focus more on the business logic rather than the repetitive parts of your code.
Example:
Let's consider a User entity that typically requires getters, setters, and other methods.
Without Lombok:-
Without Lombok, you need to manually write getters, setters, and other methods, leading to a lot of repetitive and verbose code.
With Lombok:-
4. Use Meaningful Naming Conventions
Use descriptive names for classes, methods, and variables to enhance code readability.
Example:
Class Names:
- Bad: Data, Ctrl, Manager, Info
- Good: UserData, UserController, OrderManager, CustomerInfo
Method Names:
- Bad: doTask(), handle(), process(), execute()
- Good: createUser(), getUserById(), updateOrderStatus(), sendNotification()
Variable Names:
- Bad: temp, data, val, x
- Good: userList, userId, orderDate, customerEmail
5. Handle Exceptions Gracefully
- Proper exception handling is crucial for building robust and user-friendly applications. It involves catching and handling exceptions in a way that doesn't crash the application, providing meaningful error messages to users, and logging necessary details for developers to debug.
- Spring Boot provides several ways to handle exceptions, such as using @ExceptionHandler, @ControllerAdvice, and custom exceptions.
Example:
Let's consider a scenario where we have a UserService that might throw exceptions during user creation. We will handle these exceptions gracefully using custom exceptions and @ControllerAdvice.Lets go step by step:
6. Write Unit Tests (We will explore more in the upcoming sections)
- Ensure comprehensive unit testing for all components using JUnit and Mockito.
Example:
7. Document Your Code
- Use JavaDocs to document classes, methods, and APIs for better understanding and maintenance. You can easily add Javadoc using IntelliJ's context actions. Place the caret at the method, class, or other declaration you want to document in the IntelliJ editor, press Alt + Enter, and select 'Add Javadoc' from the list to generate the Javadoc.
Example:
So? How was that? Liked it? I’m sure it was easier for you to handle since it didn't overwhelm your mind.
By following these best practices, you can write clean, efficient, and maintainable code in your Spring Boot projects. This approach not only enhances the quality of your codebase but also improves collaboration and productivity within your development team.
Exploring Code Testing in Spring Boot
We have seen a small overview of testing in the previous section. Now, let's dive deeper into the essential aspects of testing your Spring Boot applications. Testing is a crucial part of software development that ensures your code behaves as expected, catches bugs early on, and provides confidence in the stability and reliability of your application.
Spring Boot applications require thorough testing of controllers, services, repositories, and integrations. Here’s how you can set up and write tests:
Setting Up Testing in Spring Boot
- Add Testing Dependencies
- Ensure you have the necessary dependencies in your pom.xml for JUnit, Mockito, and Spring Test.
Writing Tests in Spring Boot
Unit Testing Controllers, Services, and Repositories
Unit tests isolate individual layers of your application.
2. Integration Testing
Integration tests validate that different parts of the application work together correctly.
- Explanation:
- UserControllerIntegrationTest.java: Integration tests for the UserController class.
- The test verifies that the getUserById endpoint returns the correct user data by setting up a real environment with a database.
By setting up comprehensive testing environments in Spring Boot, you can ensure your applications are robust, reliable, and maintainable. From unit tests to integration tests, these practices help catch bugs early and give you confidence in your code. Start implementing these testing strategies to build high-quality Spring Boot applications.
Optimizing Performance for Spring Boot Applications
As applications grow, performance optimization becomes crucial to ensure a smooth and responsive experience. This section explores strategies to optimize performance in Spring Boot applications.
1. Database Optimization:
- Optimize database interactions by using indexes, designing efficient queries, and implementing connection pooling. Proper indexing and query optimization can significantly improve database performance.
- How It Works:
- Indexes: Adding indexes to frequently queried columns speeds up data retrieval.
- Efficient Queries: Writing optimized queries and using joins appropriately to minimize database load.
- Connection Pooling: Reusing database connections rather than opening new ones for each request, reducing overhead.
Example:
- Code explanation:
- The @Table annotation is used to define indexes on the name column, improving the speed of queries that search by name.
2. Caching:
- Use caching mechanisms like Spring Cache to store frequently accessed data and reduce database load. Caching can drastically reduce the response times for repeat queries.
- How It Works:
- @Cacheable Annotation: Annotate methods whose results should be cached.
- Cache Manager: Configure a cache manager to manage cached data.
- Cache Store: Frequently accessed data is stored in the cache store (e.g., in-memory, Redis), reducing the need to query the database repeatedly.
Example:
- Code explanation:
- @Cacheable caches the result of getUserById method calls. @EnableCaching enables Spring's annotation-driven cache management.
3. Asynchronous Processing:
- Use asynchronous processing for tasks that can be executed in parallel, improving responsiveness and throughput. Asynchronous methods run in separate threads, freeing up the main thread for other tasks.
- How It Works:
- @Async Annotation: Annotate methods that should be executed asynchronously.
- Thread Pool Executor: Configure a thread pool executor to manage asynchronous tasks.
- Parallel Execution: Asynchronous tasks are executed in parallel threads, allowing the main application flow to continue without waiting for these tasks to complete.
Example:
- Code explanation:
- @Async makes the sendEmail method run asynchronously. @EnableAsync enables Spring’s async support. SimpleAsyncTaskExecutor is a basic thread pool executor.
4. Create Microservices:
Implementing microservices can greatly enhance the performance of Spring Boot applications, especially when managing systems with multiple features. In a monolithic architecture, scaling to meet the demands of a single feature often requires allocating additional resources to the entire application, including features that don't need it. With microservices, each feature is developed, deployed, and scaled independently. For instance, if one feature requires more resources due to increased traffic, only that service can be scaled without affecting others. This targeted scaling improves resource efficiency and overall performance. To learn more about microservices, check out our “Microservices vs Monolith” blog here.
5. Optimizing Application Configuration:
By implementing these configuration optimizations, you can significantly improve the performance and efficiency of your Spring Boot application.
Monitoring and Logging for Spring Boot Application
Monitoring and logging are essential for maintaining the health and performance of your application. They help you detect issues early, understand usage patterns, and optimize your system. In this section, we'll discuss setting up monitoring and logging for your Spring Boot backend.
Monitoring and Logging for Spring Boot
1. Spring Boot Actuator
Spring Boot Actuator provides production-ready features to help you monitor and manage your application.
2. Logging with Spring Boot
Spring Boot uses SLF4J for logging by default. You can configure it to use different logging frameworks like Logback, Log4j2, or Java Util Logging.
3. Integrating with Monitoring Tools
Integrating your Spring Boot application with monitoring tools like Prometheus, Grafana, ELK Stack, and SonarQube provides advanced monitoring, logging, and code quality analysis capabilities. Here’s a detailed look at how to integrate each of these tools:
Prometheus and Grafana: Monitor Metrics and Visualize Data
ELK Stack (Elasticsearch, Logstash, Kibana): Centralize Logs and Visualize Them
SonarQube: Analyze Code Quality and Detect Issues
An open-source platform for continuous inspection of code quality to perform automatic reviews with static analysis of code to detect bugs, code smells, and security vulnerabilities.
By integrating SonarQube into your Spring Boot projects, you can continuously monitor code quality, detect issues early, and maintain a high standard of code hygiene.
Seamless Deployment Strategies Spring Boot Applications
Deploying applications can be a seamless process if approached with the right strategy. In this guide, we’ll use React as the frontend example and a Spring Boot backend to walk you through various deployment options and best practices, ensuring your applications run smoothly in production.
Containerization with Docker
Containerization using Docker is a powerful method to deploy both React and Spring Boot applications. Docker ensures consistency across different environments and simplifies dependency management by packaging applications and their dependencies into isolated containers.
Dockerizing a Spring Boot Application
a. Create a Dockerfile
A Dockerfile is a script that contains instructions on how to build a Docker image for your application. In your Spring Boot project directory, create a file named ‘Dockerfile’ with the following content:
Explanation:
- FROM openjdk:11-jre-slim: Uses the official OpenJDK image as the base image.
- EXPOSE 8080: Exposes port 8080 for the application.
- ARG JAR_FILE=target/*.jar: Uses a build argument to specify the JAR file location.
- ADD ${JAR_FILE} app.jar: Adds the JAR file to the container.
- ENTRYPOINT ["java","-jar","/app.jar"]: Specifies the command to run the JAR file.
b. Build the Docker Image
Navigate to your project directory and run the following command to build the Docker image:
This command creates a Docker image named my-spring-boot-app using the instructions in the Dockerfile.
c. Run the Docker Container
Run the Docker container using the built image:
This command maps port 8080 of the host machine to port 8080 of the container and runs the application.
Dockerizing a React Application
1. Create a Dockerfile
In your React project directory, create a file named Dockerfile with the following content:
Explanation:
- Stage 1: Build the React application
- FROM node:14-alpine as build: Uses the official Node.js image to build the application.
- WORKDIR /app: Sets the working directory inside the container.
- COPY package.json ./: Copies the package.json file to the container.
- RUN npm install: Installs the dependencies.
- COPY . .: Copies the entire project to the container.
- RUN npm run build: Builds the React application.
- CMD ["nginx", "-g", "daemon off;"]: Starts Nginx in the foreground.
- FROM node:14-alpine as build: Uses the official Node.js image to build the application.
- Stage 2: Serve the React application using Nginx
- FROM nginx:alpine: Uses the official Nginx image to serve the application.
- COPY --from=build /app/build /usr/share/nginx/html: Copies the build output from the first stage to Nginx's HTML directory.
- EXPOSE 80: Exposes port 80 for the application.
- CMD ["nginx", "-g", "daemon off;"]: Starts Nginx in the foreground.
2. Build the Docker Image
Navigate to your project directory and run the following command to build the Docker image:
This command creates a Docker image named my-react-app using the instructions in the Dockerfile.
3. Run the Docker Container
Run the Docker container using the built image:
This command maps port 80 of the host machine to port 80 of the container and runs the application.
Running Containers with Docker Compose
Docker Compose is a tool that allows you to define and run multi-container Docker applications. It uses a YAML file to configure the application’s services. In this example, we'll use Docker Compose to run both the React and Spring Boot applications.
1. Create a docker-compose.yml file
In your project directory, create a file named docker-compose.yml with the following content:
Explanation:
- version: '3': Specifies the version of the Docker Compose file format.
- services: Defines the services (containers) that make up the application.
- backend: Defines the Spring Boot application service.
- image: my-spring-boot-app: Uses the my-spring-boot-app image.
- ports: - "8080:8080": Maps port 8080 of the host to port 8080 of the container.
- frontend: Defines the React application service.
- image: my-react-app: Uses the my-react-app image.
- ports: - "80:80": Maps port 80 of the host to port 80 of the container.
- backend: Defines the Spring Boot application service.
a. Start the Services
Run the following command to start the services defined in the docker-compose.yml file:
This command starts the containers in detached mode.
b. Verify the Deployment
Open your web browser and navigate to http://localhost:8080 for the React application and http://localhost:8080 for the Spring Boot application to verify that both applications are running correctly.
By containerizing your applications using Docker, you can ensure a consistent and portable deployment process, making it easier to manage dependencies and environment configurations.
Deployment on Cloud Platforms
Deploying applications on cloud platforms offers scalability, reliability, and ease of management. Using cloud platforms like AWS, GCP, or Azure can streamline your deployment process.
Here we are taking AWS as example
2. Deployment on Cloud Platforms
Deploying applications on cloud platforms offers scalability, reliability, and ease of management. Using cloud platforms like AWS, GCP, or Azure can streamline your deployment process.
Here we are taking AWS as example
3. Continuous Integration/Continuous Deployment (CI/CD)
Implementing Continuous Integration (CI) and Continuous Deployment (CD) pipelines ensures that your code is tested, integrated, and deployed automatically. This reduces manual intervention, minimizes the risk of errors, and helps maintain high code quality and deployment consistency. In this section, we'll cover setting up CI/CD pipelines using popular tool GitHub Actions.
CI/CD Using GitHub Actions
GitHub Actions is a powerful tool that enables you to automate workflows directly from your GitHub repository. Let's walk through setting up a CI/CD pipeline for both React and Spring Boot applications.
Steps:
- Create a GitHub repository for your project.
- In your repository, create a new directory called .github/workflows and add a YAML file for the workflow (e.g: deploy.yml).
Explanation:
- The ‘on’ section specifies the events that trigger the workflow. Here, it's triggered on pushes to the ‘main’ branch.
- The ‘jobs’ section defines the steps for the CI/CD pipeline.
- ‘checkout@v2’ checks out the repository.
- ‘setup-java@v1’ sets up JDK 11 for building the Spring Boot application.
- The ‘mvn clean install’ command builds the Spring Boot application.
- ‘setup-node@v2’ sets up Node.js for building the React application.
- The ‘npm install’ and ‘npm run build’ commands install dependencies and build the React application.
- Finally, the Docker images for both applications are built and pushed to DockerHub.
4. Integrating Git Hooks
Git hooks are scripts that run automatically at certain points in the Git workflow, such as before making a commit or pushing code to a repository. They can be used to enforce code quality and streamline development processes.
Setting Up Git Hooks:
Explanation:
- The ‘pre-commit’ hook runs before each commit.
- The script runs ‘npm run lint’ to check for linting errors.
- If linting fails, the commit is aborted, ensuring code quality before committing.
By following these strategies, you can ensure a smooth and efficient deployment process for your React and Spring Boot applications. Containerization with Docker, leveraging cloud platforms, implementing CI/CD pipelines, and integrating Git hooks will help you maintain high code quality and streamline your development workflow.
How can CodeWalnut help you build world-class, enterprise-level applications with a Java Spring Boot backend?
Building a high-quality custom application with Java Spring Boot needs careful development. You need clean maintainable code, automated tests, performance, secure coding practices and one-click deployment.
CodeWalnut, a boutique software company with top 1% talent, excels in these areas. With CodeWalnut you get a quality talent pool, strong engineering practices and agile collaboration.
Whether you're looking to migrate an existing system to a modern tech stack or build a new enterprise solution from scratch, CodeWalnut brings you engineering excellence from the get go.
Talk to a helpful CodeWalnut architect, discuss your needs and win back your peace of mind.
Key TakeAways
Clean Code Practices: Implementing clean code principles ensures your codebase is maintainable, readable, and scalable.
Performance Optimization: Optimize the performance of your Spring Boot application to ensure a fast and responsive user experience.
Comprehensive Monitoring and Logging: Effective monitoring and logging are crucial for maintaining the health and performance of your applications.
Seamless Deployment: Deploy your applications efficiently using containerization, CI/CD pipelines, and cloud services.
FAQ
1. What is the advantage of using Spring Boot for backend development?
Spring Boot simplifies backend development with its efficiency and ease of use. It provides a robust framework for building production-ready applications quickly by reducing boilerplate code, offering built-in features for security, data access, and more, and promoting best practices for scalability and performance.
2. How can I optimize the performance of my Spring Boot application?
Optimize Spring Boot performance by leveraging techniques such as caching, database indexing, and efficient query optimization. Additionally, using Spring Boot Actuator to monitor and manage application performance can help identify bottlenecks and improve efficiency.
3. What monitoring tools can I use for my Spring Boot application?
Monitor your Spring Boot app using Spring Boot Actuator. It provides production-ready features like health checks, metrics, and endpoint monitoring, ensuring your application's health and performance are tracked effectively. Integration with tools like Prometheus and Grafana can further enhance monitoring capabilities.
4. Why is containerization beneficial for deploying Spring Boot applications?
Containerization ensures consistent deployment across different environments. Docker allows you to package your Spring Boot application with all its dependencies, making deployments easier and more reliable, whether on-premise or in the cloud. This approach minimizes environment-specific issues and simplifies scaling.
5. How can I set up a CI/CD pipeline for my Spring Boot applications?
Use tools like GitHub Actions or Jenkins to automate your build, test, and deployment processes. A CI/CD pipeline for Spring Boot applications helps streamline development workflows, ensures rapid and reliable releases, and facilitates continuous integration and deployment practices.
6. What role does Lombok play in Java Spring Boot development?
Lombok reduces boilerplate code in Java classes by providing annotations that generate common methods like getters, setters, and constructors automatically. This improves code readability and maintainability without sacrificing performance, making development faster and more efficient.
7. How can I ensure code quality in my Spring Boot application?
Utilize SonarQube for code quality analysis. SonarQube performs static code analysis, detecting bugs, security vulnerabilities, and code smells. This ensures that your Spring Boot applications meet high standards of quality and maintainability, leading to more reliable and secure code.
8. What are Git hooks, and how can they benefit my Spring Boot development workflow?
Git hooks are scripts that automate tasks before commits and pushes. They can enforce coding standards, run tests, and perform other checks, ensuring code quality and preventing issues from entering your repository. Incorporating Git hooks into your workflow helps maintain a clean and consistent codebase.
Get in touch - Our team has developed scalable solutions for enterprises and has a Crunch rating of 4.9⭐.
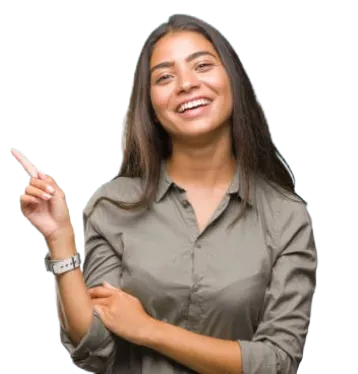
Experience coding prowess firsthand. Choose CodeWalnut to build a prototype within a week and make your choice with confidence.
Accelerate your web app vision with CodeWalnut. In just a week, we'll shape your idea into a polished prototype, powered by Vercel. Ready to make it real? Choose us with confidence!
Dreaming of a powerful web app on Heroku? Let CodeWalnut bring it to life in just one week. Take the leap and trust us to deliver with confidence!